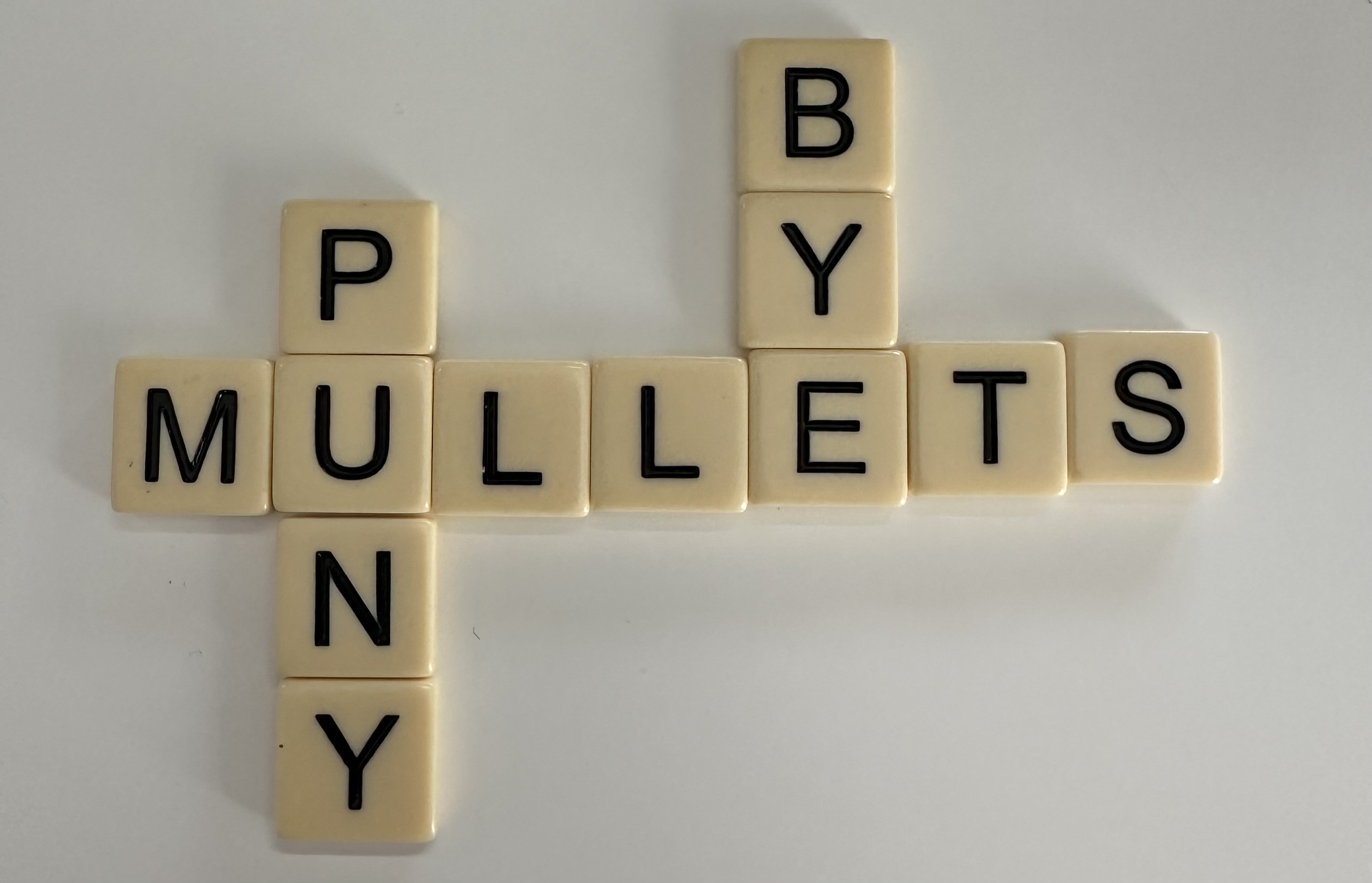
Q-Less For All
I’m a big fan of word games. I’ll play Scrabble or Bananagrams whenever I can find a willing partner (finding a willing partner is the hard part). So when I saw an ad for Q-Less, a solo word game based on dice, I knew I had to try it out.
Q-Less Mechanics
A game of Q-Less starts by rolling the 12 letter-covered dice. Each roll gives you a random set of 12 letters. Using all 12 letters, you have to spell words that connect just like in Scrabble or Bananagrams.
My first thought was: can I play this game with the Scrabble or Bananagrams tiles that I already have?
Dice-Less Q-Less
The dice in Q-Less provide 2 important things to the game:
- The set of letters that are possible to roll
- A way to randomly select from that set of letters (rolling the dice)
If I could find the set of letters possible to roll, I could write a simple program to “roll” the dice for me and generate a set of 12 letters which I could then pick out from my set of letter tiles (provided I had enough tiles).
First I found the list of dice and their face values here. I later verified this list to be accurate with my own Q-Less dice (yes, I eventually bought the game for myself).
Necessary Tiles
Once I knew what the dice looked like, I needed to verify if Scrabble and/or Bananagrams had the tiles sufficient to support all possible rolls. I found the number of each letter tile in Scrabble and Bananagrams conveniently listed here. I then wrote a simple script to calculate the number of each letter tile needed to cover every possible Q-Less roll, shown in the following table:
A | B | C | D | E | F | G | H | I | J | K | L | M |
2 | 3 | 2 | 3 | 2 | 2 | 2 | 2 | 2 | 1 | 2 | 3 | 2 |
N | O | P | Q | R | S | T | U | V | W | X | Y | Z |
3 | 3 | 2 | 0 | 3 | 2 | 3 | 1 | 1 | 2 | 1 | 2 | 1 |
const dieSides = 6;
const dice = [
"MMLLBY",
"VFGKPP",
"HHNNRR",
"DFRLLW",
"RRDLGG",
"XKBSZN",
"WHHTTP",
"CCBTJD",
"CCMTTS",
"OIINNY",
"AEIOUU",
"AAEEOO"
]
// print out stats on how many of each letter tile is needed
// answers the question "how many dice does this letter show up on"
function letterStats()
{
const numLetters = 26;
const charCodeA = 65;
let alphabet = new Array(numLetters).fill(0);
dice.forEach( die =>
{
let lettersSeen = new Set();
for (let i=0; i < dieSides; i++)
{
let alphaIndex = die.charCodeAt(i) - charCodeA;
lettersSeen.add(alphaIndex);
}
lettersSeen.forEach( alphaIndex =>
{
alphabet[alphaIndex]++;
});
});
for (let i=0; i < numLetters; i++)
{
let letter = String.fromCharCode(charCodeA + i);
console.log(letter + ": " + alphabet[i]);
}
}
So indeed, the tiles needed for Q-Less are a subset of the tiles included with Scrabble and Bananagrams.
Rolling the Dice
From here, it was trivial to write some JavaScript to generate my Q-Less “roll”:
Playing the Game
Using the table above, I set aside only the set of tiles needed to play Q-Less from my Bananagrams tiles, into a separate bag. This makes it slightly easier to dig through the tiles for each roll. Then I roll the dice using the script above, pick out those letters from my tiles, and play a round of Dice-Less Q-Less.
Conclusion
While I had fun with this little challenge and enjoyed being able to try before I buy, I did end up purchasing Q-Less for myself and for a friend. If you do end up using this method to play the game, and you enjoy it, I would definitely recommend supporting the creator of the game and purchasing it. Rolling the dice is actually a lot more satisfying than picking out the tiles anyway.